How to Build a Simple Tip Calculator Shortcut with Jellycuts
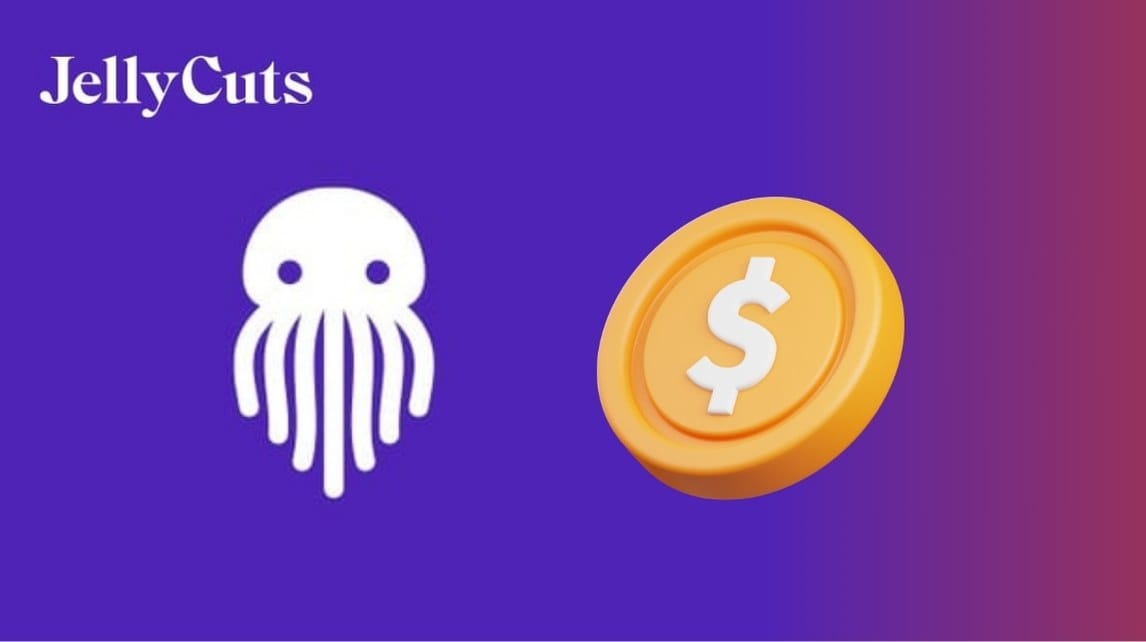
In this tutorial, we’ll walk through the process of creating a simple yet effective Tip Calculator using Jellycuts, which allows us to create useful Shortcuts more efficiently. Our process is to ask the user for the total bill amount and tip percentage, calculate the tip, and then display both the tip and the total bill with the tip included.
By the end of this tutorial, you’ll be able to build a fully functional tip calculator that you can use in your daily life. Let’s get started!
What You'll Need:
- Jellycuts installed on your device or access to Jellycuts IDE.
- Jellycuts Helper correctly added in your shortcuts library
Step 1: Prompt the User for Input
First, we need to ask the user for two important pieces of information: the total bill amount and the tip percentage. Jellycuts allows us to use the askForInput
function to gather this data from the user.
Here’s how we set up the prompt:
// Ask the user for the total bill amount, specifying optional parameters to avoid warnings
askForInput(prompt: "Total Bill", type: Number, default: 0, allowDecimal: true, allowNegative: false) >> billTotalInput
// Store the total bill amount in a variable
var billTotal = billTotalInput
Explanation:
askForInput
: This function is used to display a prompt to the user. Here, we ask for the Total Bill as a number. We also set some optional parameters to avoid any potential warnings:default: 0
: Sets the default value to 0.allowDecimal: true
: Allows decimal numbers (for prices like $12.99).allowNegative: false
: Ensures no negative numbers are input.
After getting the user’s input, we store it in the variable billTotal
.
We repeat this process to ask for the Tip Percent:
// Ask the user for the tip percentage, specifying optional parameters
askForInput(prompt: "Tip Percent", type: Number, default: 0, allowDecimal: true, allowNegative: false) >> tipPercentInput
// Store the tip percentage in a variable
var tipPercent = tipPercentInput
Step 2: Calculate the Tip
Now that we have the total bill amount and the tip percentage, we can calculate the tip itself. This is done by dividing the tip percentage by 100 (to convert it into a decimal) and then multiplying it by the total bill amount.
// Calculate the tip value (percentage / 100 * total bill)
calculate(input: "${tipPercent} / 100") >> tipDecimal
calculate(input: "${tipDecimal} * ${billTotal}") >> tipValue
Explanation:
- The first calculation converts the tip percentage into a decimal (
tipPercent / 100
). - The second calculation multiplies the decimal by the total bill to get the tip value.
Step 3: Round the Tip Value
For a better user experience, we’ll round the tip to two decimal places using the round
function. This ensures the output is a nicely formatted currency value.
// Round the tip value to two decimal places
round(number: tipValue, roundTo: Hundredths) >> roundedTip
Explanation:
- The
round
function rounds the tipValue to two decimal places, which makes sense for monetary values.
Step 4: Calculate the Total Bill Including the Tip
Now, we calculate the total amount to be paid by adding the rounded tip to the original bill amount.
// Calculate the total bill with the tip included
calculate(input: "${billTotal} + ${roundedTip}") >> totalWithTip
Explanation:
- We simply add the
billTotal
androundedTip
to get the total amount to be paid, including the tip.
Step 5: Display the Result
Finally, we display the result to the user using the showResult
function. This will show both the calculated tip and the total amount, formatted neatly.
// Display the result showing the tip and the total amount to be paid with the tip
showResult(text: "Tip: $${roundedTip} Total bill with tip: $${totalWithTip}")
Explanation:
- The
showResult
function presents the final message to the user, including both the Tip and the Total bill with the tip.
Full Code for the Tip Calculator
import Shortcuts
#Color: grayBlue, #Icon: dollarSign
// Ask the user for the total bill amount, specifying optional parameters to avoid warnings
askForInput(prompt: "Total Bill", type: Number, default: 0, allowDecimal: true, allowNegative: false) >> billTotalInput
// Store the total bill amount in a variable
var billTotal = billTotalInput
// Ask the user for the tip percentage, specifying optional parameters
askForInput(prompt: "Tip Percent", type: Number, default: 0, allowDecimal: true, allowNegative: false) >> tipPercentInput
// Store the tip percentage in a variable
var tipPercent = tipPercentInput
// Calculate the tip value (percentage / 100 * total bill)
calculate(input: "${tipPercent} / 100") >> tipDecimal
calculate(input: "${tipDecimal} * ${billTotal}") >> tipValue
// Round the tip value to two decimal places
round(number: tipValue, roundTo: Hundredths) >> roundedTip
// Calculate the total bill with the tip included
calculate(input: "${billTotal} + ${roundedTip}") >> totalWithTip
// Display the result showing the tip and the total amount to be paid with the tip
showResult(text: "Tip: $${roundedTip} Total bill with tip: $${totalWithTip}")
Final Thoughts
You’ve now built a complete tip calculator using Jellycuts! This shortcut can be easily triggered on your iOS device to quickly calculate tips and totals whenever you’re at a restaurant or paying for a service. Not only does this make your life easier, but it’s also a great way to get familiar with Jellycuts' functionality.
This project can also be extended with more features, like adding different rounding modes, handling tax calculations, or even allowing for multiple bill splitting.